Generate Unique Random Key In Java
- Unique Random Numbers In Excel
- Java Random Number 1 100
- Generate Unique Random Key In Java Free
- Java Random Between Two Numbers
- Random String Generator. This form allows you to generate random text strings. The randomness comes from atmospheric noise, which for many purposes is better than the pseudo-random number algorithms typically used in computer programs.
- KeyGenerator objects are reusable, i.e., after a key has been generated, the same KeyGenerator object can be re-used to generate further keys. There are two ways to generate a key: in an algorithm-independent manner, and in an algorithm-specific manner.
I need to generate a number, based on the primary key of an table, which will be used as an external reference (e.g. to label a product, or generate a barcode).
The number will be stored on database and later used to retrieve the actual record it was derived from.
The number has to be of a fixed length, say max 8 characters to minimize the length of the barcode.
Are there any APIs I can use to generate this number?
The solution need not imperatively derive the number form the primary key; I only need to ensure it will be unique across all records.
Thanks,
Shehzaad
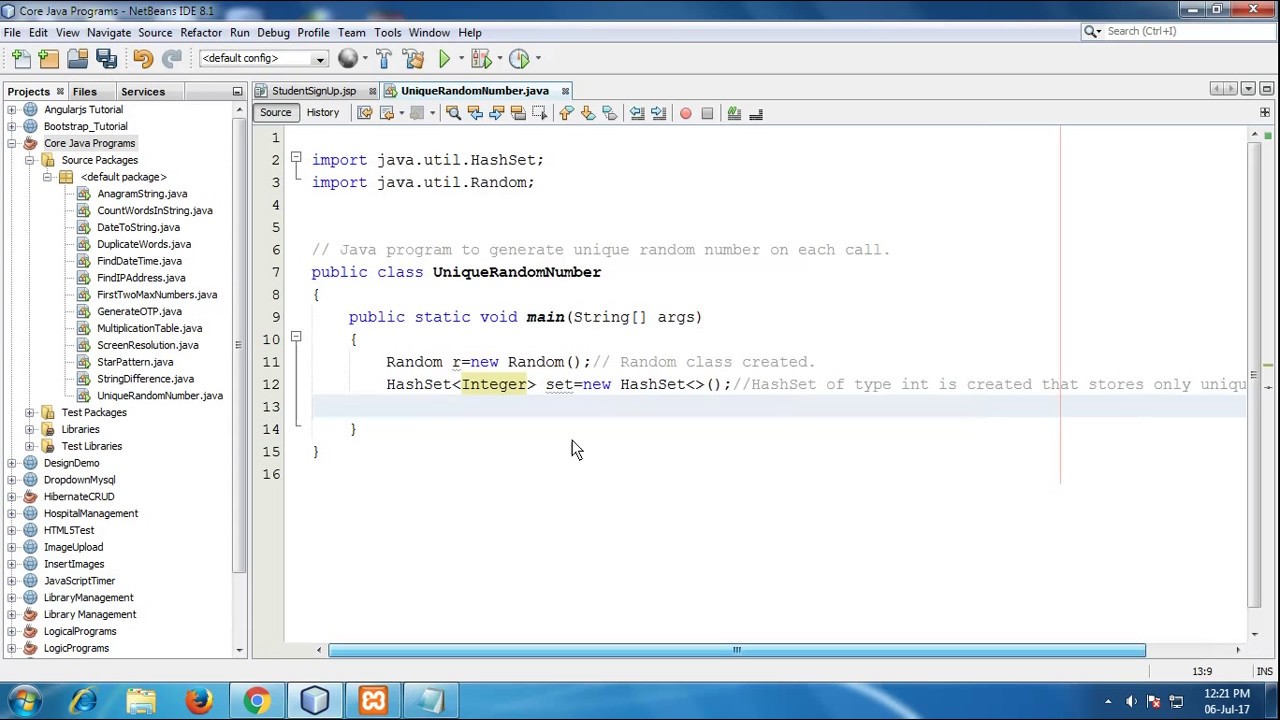
Why don’t you simply use the key from the table? DBMSs are designed to work out that sort of thing quickly.
Below is a way I used to generate unique number always. Random function generates number and stores it in textfile then next time it checks it in file compares it and generate new unique number hence in this way there is always a new unique number. If Java 5 is not available, then there are other more laborious ways to generate unique ids (see below). Style 2 - SecureRandom and MessageDigest. The following method uses SecureRandom and MessageDigest: upon startup, initialize SecureRandom (this may be a lengthy operation) when a new identifier is needed, generate a random number using.
Notwithstanding of the detail that Microsoft had launched MS office 2007, Office 2010, Office 2013 and office 2016. Excel 2003 product key generator. But office 2003 is motionless in the request.You can download Office 2003 for Windows XP and 2000.
Campbell Ritchie wrote:How can you be sure the numbers will be unique?
Well, if it's a primary key, the chances are that it has to be (although some databases do allow tables with no key).
@shehzaad: First off, do you actually need a number? Or will a code do?
Second: is the barcode itself a numeric code? 8 digits seems awfully short for any sort of standard barcode (eg, a UPC or SKU). If so, I'd just use it and not worry about the length (a Javalong can hold numbers up to 18 digits).
Winston
'Leadership is nature's way of removing morons from the productive flow' - Dogbert
Articles by Winston can be found here
some precisions:
1) I need to keep the length of the reference to a minimum, so that the 'barcode lines' generated are also a minimum. (I am using code-39)
I tried using a hash (md5, sha-1) of the primary key, but this generates 32-character long hex strings.
2) Any of the characters allowed by code-39 will do; I do not have to stick to purely numbers.
3) I have to stick to a java solution, not to a db-tied one.
shehzaad goonoo wrote:The number will be stored on database and later used to retrieve the actual record it was derived from.
shehzaad goonoo wrote:I tried using a hash (md5, sha-1) of the primary key, but this generates 32-character long hex strings.
If this is one of your requirements, then using a hash code is not going to work.
Hashing algorithms such as MD5 and SHA-1 are one way. You can calculate the hash over some data, but it is impossible to get back the original data if you have only the hash. So if you need to be able to find the record back later, then that's not possible when you use a hash. You'll need some algorithm that is reversible.
shehzaad goonoo wrote:1) I need to keep the length of the reference to a minimum, so that the 'barcode lines' generated are also a minimum. (I am using code-39)
I tried using a hash (md5, sha-1) of the primary key, but this generates 32-character long hex strings.
So, wait a minute..
Are you trying to find a way of generating a code-39 barcode that will cover your entire stock (or whatever) in 8 characters? because that's a completely different question. And the answer to that is almost certainly 'yes'.
Or are you trying to find a way of condensing a code-39 barcode to a unique 8-digit number? The answer to that is most assuredly 'NO'.
Or are you looking for something in between (like a hashcode) - an 8-digit number that is probably unique. The answer to that is, absolutely, 'YES'.
I think you need to tell us exactly what it is you want, and then move on from there.
It should probably also be pointed that 'keep the length of the reference to a minimum, so that the 'barcode lines' generated are also a minimum' is a very artificial (and arbitrary) restriction that has no basis in normal stock-keeping, so you might want to revise it.
Winston
'Leadership is nature's way of removing morons from the productive flow' - Dogbert
Articles by Winston can be found here
- 1
To put it simply:
1. as input, I have a table's primary key
2. as output, I want another unique number (It is not a requirement for it to be based on the primary key or not, as long as it stays unique across the rows of the table) of a reasonable length, say 16 digits max.
3. It has to be a Java solution
Thanks
shehzaad goonoo wrote:1. as input, I have a table's primary key
2. as output, I want another unique number (It is not a requirement for it to be based on the primary key or not, as long as it stays unique across the rows of the table) of a reasonable length, say 16 digits max.
3. It has to be a Java solution.
OK, now you're getting the idea. Some questions back to you (based on your numbering):
1:
(a) How long is the primary key?
(b) Is it numeric or alphanumeric?
(c) Does it contain any redundancy (for example, a prefix which is the same for all items)?
2:
(a) Does it have to be a number? Or can it be a code?
The simplest form of unique identifier is a sequence number and, unless you have a computer bigger than my apartment block, that will almost certainly fit into 16 digits. You may however, need a way to persist the 'last number used' value.
Java also provides a UUID class, which is designed specifically for creating unique IDs. In fact, it is not guaranteed to produce a unique ID, but the chances of it doing so are so small as to be basically nil. Also, the only automated form is a time-based UUID. Unfortunately, a UUID consists of two longs (ie, 32 hexadecimal digits or 36 decimals) so it's probably too long for your purposes.
Finally, you could generate one yourself. One possible suggestion:
42 bits contains enough space to store approximately 135 years worth of milliseconds.
10 bits contains enough space to store the fraction of a millisecond that makes a microsecond.
which leaves 11 bits left over to store a random number between 0 and 2047.
Highly likely to be unique (NOT guaranteed though), fits in a long (18 digits), and could be generated easily and quickly from System.currentTimeMillis(), System.nanotime() and Random.nextInt(2048) (or indeed, simply cycling a sequence). Furthermore, if you put it together properly, it will sort in the order that IDs were created (or very close).
Personally, I'd look at a sequence number first though.
Winston
'Leadership is nature's way of removing morons from the productive flow' - Dogbert
Articles by Winston can be found here
But like every other 'temporary solution', it is in production since 3 weeks now! I am therefore sharing the 'solution' which went into prod; it would be great to have your views on it, as well as a heads-up on any imminent crash!
Basically, a 9-digit 'code' is now being generated using Java's Random class, passing as the seed, the primary-key of a freshly created barcode row:
(Your current code doesn't guarantee uniqueness, but you're very probably aware of that.)
I need to generate a unique hash key for all users that are registering on my application and send them a confirmation email link that will activate their account. I'm considering to use UUID for generating the unique id. I'm trying to generate it based on the users email address. I would like to know if that would be a correct approach??
I'm trying something like:
I would like to know if this is this solid, secure enough or do I have to do more and use a Crypto kind of an implementation to acheive this??
SCJP 1.4, SCWCD 1.4 - Hints for you, Certified Scrum Master
Did a rm -R / to find out that I lost my entire Linux installation!
MD5
SHA1
UUID
SCJP 1.4, SCWCD 1.4 - Hints for you, Certified Scrum Master
Did a rm -R / to find out that I lost my entire Linux installation!
MD5 and SHA1 are hashing algorithms, so they do not guarantee that you get a unique output for any input.
UUID is a universally unique identifier. It is not a hashing algorithm.
Jothi Shankar Kumar wrote:
Unique Random Numbers In Excel
This will not work and will most likely give you an IllegalArgumentException. You cannot put any arbitrary string into this method - it has to be a string that represents an UUID (as returned by UUID.toString()). It's not a method that generates a unique ID based on arbitrary input.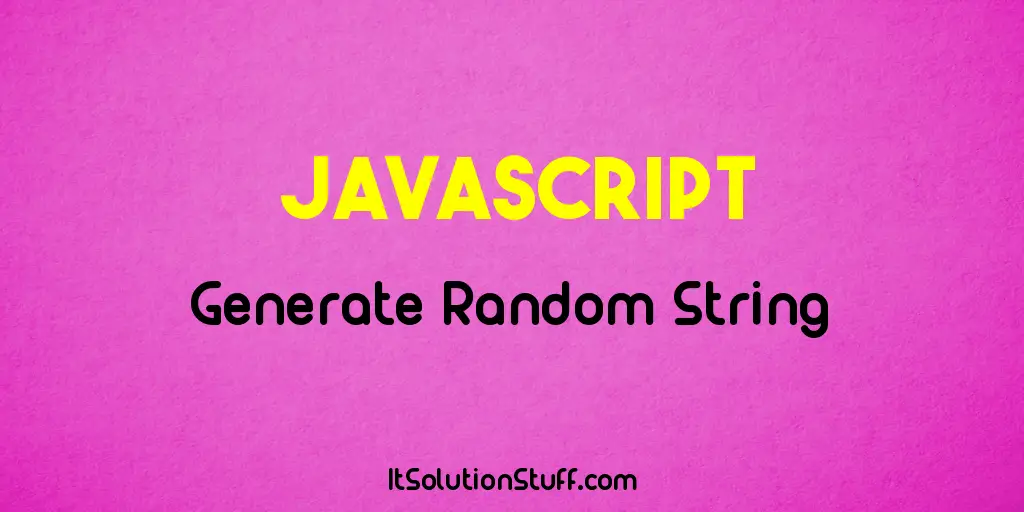
If you're looking for something that creates a unique ID based on arbitrary input - such a method can't exist in principle. (If you invent a method that does this, then you have discovered the ultimate compression algorithm and you'll win a Nobel prize in information theory.. See Jan Sloot).
SCJP 1.4, SCWCD 1.4 - Hints for you, Certified Scrum Master
Did a rm -R / to find out that I lost my entire Linux installation!
You can create random unique IDs using the UUID class:
But, as I explained above, you cannot create unique identifiers based on some arbitrary input such as the user's e-mail address.
Jothi Shankar Kumar wrote:So how could I acheive a unique key for my case here?
Hash and unique key are two different things, but to answer your question..
If you want to generate a key for a single application, then the UUID class should be fine. If it is a group of applications, meaning that you can be generating it from many applications and on many machines -- then you probably need to create your own class that uses the host, pid, and probably system time. Regardless, it is probably best to use a service (such as a database) to generate the id.
Henry
Java Random Number 1 100
Books: Java Threads, 3rd Edition, Jini in a Nutshell, and Java Gems (contributor)
Jesper Young wrote:Generate a unique ID, then store in a database somewhere which ID is associated with which user. When the user tries to activate the account using the ID, look the user's information up in the database.
You can create random unique IDs using the UUID class:
But, as I explained above, you cannot create unique identifiers based on some arbitrary input such as the user's e-mail address.
I understand what you meant here. But how unique is the randomUUID() generator?
SCJP 1.4, SCWCD 1.4 - Hints for you, Certified Scrum Master
Did a rm -R / to find out that I lost my entire Linux installation!
For practical purposes, you don't need to worry that the generated IDs could be non-unique.
SCJP 1.4, SCWCD 1.4 - Hints for you, Certified Scrum Master
Did a rm -R / to find out that I lost my entire Linux installation!
I'm not sure what you mean by 'somewhat convincing'. I went to the page, and it's not an argument, but an explanation.
John.
SCJP 1.4, SCWCD 1.4 - Hints for you, Certified Scrum Master
Did a rm -R / to find out that I lost my entire Linux installation!
Generate Unique Random Key In Java Free
posted 10 years agoJothi Shankar Kumar wrote:I need to generate a unique hash key for all users that are registering on my application and send them a confirmation email link that will activate their account. I'm considering to use UUID for generating the unique id. I'm trying to generate it based on the users email address. I would like to know if that would be a correct approach??
No it's not. The best way is to keep a global counter (starting at 0). When a user registers he/she gets the counter number as id, and then you increment the counter by 1.
In this way you're 100% sure each user gets a unique id. There is not other way to ensure that really.
Also, from a principle standpoint, the id's will be random. They're random in the sense that no user can know what number they're going to get.
Ulrika Tingle wrote:
Jothi Shankar Kumar wrote:I need to generate a unique hash key for all users that are registering on my application and send them a confirmation email link that will activate their account. I'm considering to use UUID for generating the unique id. I'm trying to generate it based on the users email address. I would like to know if that would be a correct approach??
No it's not. The best way is to keep a global counter (starting at 0). When a user registers he/she gets the counter number as id, and then you increment the counter by 1.
In this way you're 100% sure each user gets a unique id. There is not other way to ensure that really.
But you have to synchronize the counter, or you will run into problems in a multi-threaded environment. AtomicLong can help out here to do the hard work, with its incrementAndGet() and getAndIncrement() methods, the synchronized versions of ++l and l++.
SCJP 1.4 - SCJP 6 - SCWCD 5 - OCEEJBD 6 - OCEJPAD 6
How To Ask QuestionsHow To Answer Questions
Java Random Between Two Numbers
posted 10 years agoWhy will I create a counter starting at 0 and increment that for each user when I store the user details in the database. Ofcourse I have the primary key. Don't I?
SCJP 1.4, SCWCD 1.4 - Hints for you, Certified Scrum Master
Did a rm -R / to find out that I lost my entire Linux installation!
It doesn't need to be unique if they send their email address too.